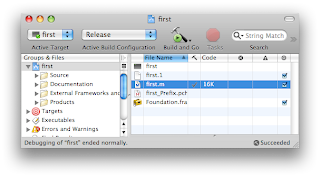
My thinking is that it would be nice to develop the code under XCode and then just compile for Linux if I can figure out where the frameworks diverge and keep to compatible code.
Here's a quick start guide for Fedora Core 5 assuming that you already have the basic gcc (I'm sure it's basically the same on others):
- sudo yum install gcc-objc
- wget ftp://ftp.gnustep.org/pub/gnustep/core/gnustep-startup-0.18.2.tar.gz
- tar xzf gnustep-startup-0.18.2.tar.gz
- cd gnustep-startup-0.18.2
- sudo ./InstallGNUstep
- Edit your ~/.bash_profile to add the line . /usr/GNUstep/System/Library/Makefiles/GNUstep.sh
- (note the leading dot in the line above)
Here is a little test program, called first.m:
#import <foundation/foundation.h>
int main(int argc, char** argv, char** env)
{
NSLog(@"Hello");
NSAutoreleasePool *pool = [[NSAutoreleasePool alloc] init];
NSMutableArray * array = [NSMutableArray new];
[array addObject: [NSString stringWithString:@"one"]];
[array addObject:[NSString stringWithString:@"two"]];
[array addObject:[NSString stringWithString:@"three"]];
[array addObject:[NSString stringWithString:@"four"]];
[array addObject:[NSString stringWithString:@"five"]];
[array addObject: @"six"];
NSEnumerator * e = [array objectEnumerator];
NSString * string;
while ((string = [e nextObject]))
{
NSLog(string);
}
[pool release];
return 0;
}
Create a file called GNUmakefile:
include $(GNUSTEP_MAKEFILES)/common.make
TOOL_NAME = first
first_OBJC_FILES = first.m
include $(GNUSTEP_MAKEFILES)/tool.make
Then make and run:
[marksp@homelinux first]$ make
Making all for tool first...
Compiling file first.m ...
Linking tool first ...
[marksp@homelinux first]$ obj/first
2007-11-04 04:38:40.246 first[1257] Hello
2007-11-04 04:38:40.276 first[1257] one
2007-11-04 04:38:40.277 first[1257] two
2007-11-04 04:38:40.278 first[1257] three
2007-11-04 04:38:40.278 first[1257] four
2007-11-04 04:38:40.279 first[1257] five
2007-11-04 04:38:40.279 first[1257] six
The same code can be built in Xcode and will run on the mac.
One immediate incompatibility I ran into was the starter code for a Foundation command line tool ended with [pool drain] instead of [pool release] - a little DTS humour no doubt!
No comments:
Post a Comment