A remote control radio is a wonderful thing, I wish there were more around. You can listen to your own signal from far away to see how it really sounds and if the remote receiver is in a quiet location with a decent antenna it might be much better than listening from your home QTH.
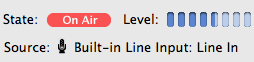
Initially I used an external USB audio input device but after about 18 hours the sound deteriorated. I think there's a bug in the Mac's USB audio chain somewhere.
For the web interface I chose a very simple cgi using python.
Unfortunately I can't really offer this up to the world as I pay for upload on my internet connection and I'm already on target to run out this month.
I hope others can build on this and set up some more remote receivers around the place.
Here's the little python cgi:
#!/usr/bin/env python
# By VK2TPM Peter Marks http://marxy.org
# You are free to use this for any purpose.
#
# Thanks to df4or.de for notes on CI-V here:
# http://www.plicht.de/ekki/civ/civ-p31.html
#
# The BCD utilities come from
# Rigserve by Martin Ewing
# http://sourceforge.net/projects/rigserve
#
import serial
import time
import cgi
import sys
import time
import string
sys.stderr = sys.stdout
SERIAL_DEV = "/dev/cu.PL2303-000013FD"
SERIAL_BAUD = 4800
INTRO = "\xfe"
TO_ADDR = "\x70"
FROM_ADDR = "\xe0"
SET_OPERATING_FREQ = "\00"
SET_OPERATING_MODE = "\x06"
READ_OPERATING_FREQ = "\x03"
READ_OPERATING_MODE = "\x04"
EOM = "\xfd"
STREAM_URL = "http://XXXXXXXXXXXXX/listen.m3u"
SCRIPT = "/cgi-bin/radio.py"
def main():
log = open("log.txt", "w")
log.write("started %s\n" % time.ctime())
ser = serial.Serial(SERIAL_DEV, SERIAL_BAUD, timeout=1)
form = cgi.FieldStorage()
if form.has_key('frequency'):
freq = form.getvalue("frequency")
log.write("freq = %s\n" % freq)
setFrequency(ser, float(freq) * 1000)
if form.has_key('mode'):
mode = form.getvalue("mode")
log.write("mode = %s\n" % mode)
setMode(ser, mode)
else:
log.write("no form submit\n")
freq = getFrequency(ser)
mode = getMode(ser)
if mode == "LSB":
mode1 = "SELECTED"
mode2 = ""
mode3 = ""
elif mode == "USB":
mode1 = ""
mode2 = "SELECTED"
mode3 = ""
elif mode == "AM":
mode1 = ""
mode2 = ""
mode3 = "SELECTED"
htmlTemplate = """<html><head>
<title>VK2TPM</title>
<style>body,td,a,p{font-family:arial,sans-serif}</style>
</head><body>
<h1>VK2TPM Web controlled radio</h1>
<form action="$script">
<table>
<tr><td>Frequency:</td><td><input name="frequency" value="$freq">Hz
<a href="$script?frequency=$freqDown&Submit=Submit">-5</a>
<a href="$script?frequency=$freqUp&Submit=Submit">+5</a> </td></tr>
<tr><td>Mode:</td><td><SELECT NAME="mode">
<OPTION VALUE="LSB" $lsbSelected>LSB
<OPTION VALUE="USB" $usbSelected>USB
<OPTION VALUE="AM" $amSelected>AM
</SELECT></td></tr>
</table>
<input name="Submit" type=submit value="Submit"> <a href="$script">Refresh</a>
</form>
<a href="$script?frequency=3700&mode=LSB">3700 LSB</a> |
<a href="$script?frequency=3670&mode=AM">3670 AM</a> |
<a href="$script?frequency=3600&mode=LSB">3600 SSB</a> |
<a href="$script?frequency=576&mode=AM">576 AM</a> |
<a href="$script?frequency=11750&mode=AM">11750 AM</a> | <br />
<a href="$script?frequency=5643&mode=AM">5643 AM</a> |
<a href="$script?frequency=8867&mode=AM">8867 AM</a> |
<a href="$script?frequency=4426&mode=USB">4426 USB</a> |
<a href="$script?frequency=8176&mode=USB">8176 USB</a> | <br />
<p>Click to listen to the stream <a href="$streamUrl">here</a>.</p>
This receiver is connected to a 40/80m trap dipole so is best around 3500 and 7000.<br />
The stream is buffered by a few seconds so don't panic after you change something.<br />
After some tidying up, I'll publish the source on the <a href="http://marxy.org">blog</a>
</body></html>
"""
template = string.Template(htmlTemplate)
html = template.substitute({ "freq": str(freq),
"freqDown": str(freq - 5),
"freqUp": str(freq + 5),
"script": SCRIPT,
"streamUrl": STREAM_URL,
"lsbSelected": mode1,
"usbSelected": mode2,
"amSelected": mode3})
print "Content-type: text/html\n\n"
print html
ser.close()
log.close()
def test():
print "started"
ser = serial.Serial(SERIAL_DEV, SERIAL_BAUD, timeout=1)
print getFrequency(ser)
print getMode(ser)
setFrequency(ser, 3670 * 1000) # Hz
ser.close()
def setFrequency(ser, freq):
fs = "%010d" % int(freq)
print fs
out = bcd4(int(fs[8]),int(fs[9]),int(fs[6]),int(fs[7]))
out += bcd4(int(fs[4]),int(fs[5]),int(fs[2]),int(fs[3]))
out += bcd2(int(fs[0]),int(fs[1]))
print out
sendStr = INTRO + TO_ADDR + FROM_ADDR + SET_OPERATING_FREQ
for byte in out:
sendStr += chr(byte)
sendStr += EOM
ser.write(sendStr)
echo = ser.read(len(sendStr))
print "got reply of %d chars" % len(echo)
def getFrequency(ser):
sendStr = INTRO + TO_ADDR + FROM_ADDR + READ_OPERATING_FREQ + EOM
ser.write(sendStr)
echo = ser.read(len(sendStr))
print "got reply of %d chars" % len(echo)
if not expectChar(ser.read(), INTRO): return
if not expectChar(ser.read(), INTRO): return
if not expectChar(ser.read(), FROM_ADDR): return
if not expectChar(ser.read(), TO_ADDR): return
byte = "0"
result = ""
while byte != EOM:
byte = ser.read()
print "%02x" % ord(byte),
result += byte
print "got EOM byte"
frequency = 0.0
if len(result) > 0:
f=0
for k in [10,11,8,9,6,7,4,5,2,3]:
f=10*f + nib(result,k)
frequency = (float(f) / 1000)
return frequency
def getMode(ser):
sendStr = INTRO + TO_ADDR + FROM_ADDR + READ_OPERATING_MODE + EOM
ser.write(sendStr)
echo = ser.read(len(sendStr))
print "got reply of %d chars" % len(echo)
if not expectChar(ser.read(), INTRO): return
if not expectChar(ser.read(), INTRO): return
if not expectChar(ser.read(), FROM_ADDR): return
if not expectChar(ser.read(), TO_ADDR): return
byte = "0"
result = ""
while byte != EOM:
byte = ser.read()
print "%02x" % ord(byte),
result += byte
print "got EOM byte"
mode = "XXX"
if result[1] == "\x00":
mode = "LSB"
elif result[1] == "\x01":
mode = "USB"
elif result[1] == "\x02":
mode = "AM"
elif result[1] == "\x03":
mode = "CW"
elif result[1] == "\x04":
mode = "RTTY"
elif result[1] == "\x05":
mode = "FM"
elif result[1] == "\x06":
mode = "Wide FM"
elif result[1] == "\x07":
mode = "CW-R"
elif result[1] == "\x08":
mode = "RTTY-R"
elif result[1] == "\x11":
mode = "S-AM"
return mode
def setMode(ser, mode):
sendStr = INTRO + TO_ADDR + FROM_ADDR + SET_OPERATING_MODE
if mode == "LSB":
sendStr += "\x00"
elif mode == "USB":
sendStr += "\x01"
elif mode == "AM":
sendStr += "\x02"
sendStr += EOM
ser.write(sendStr)
echo = ser.read(len(sendStr))
print "got reply of %d chars" % len(echo)
# non-reversed
def bcd4(d1,d2,d3,d4): return (16*d1+d2, 16*d3+d4)
# pack 2 BCD digits
def bcd2(d1,d2): return ( (16*d1+d2), )
# get a 4-bit nibble (digit) from a nibble string
def nib(s,i):
k = ord(s[i/2])
if i%2 == 0: k = k >> 4
return k & 0xf
def expectChar(byte, expected):
"""Return true if we got what we expected"""
if byte == expected:
print "good %02x" % (ord(expected)),
return True
else:
print "wanted %02x unexpected %02x" % (ord(expected), ord(byte)),
return False
main()
2 comments:
Hi Peter,
Found your nice Python script for ICOM rigs. I get some error running on WinXP. What version of Python did you use?
/Jens, SM6AFV
What error do you get? I'm using python 2.5 or 2.6.
Post a Comment